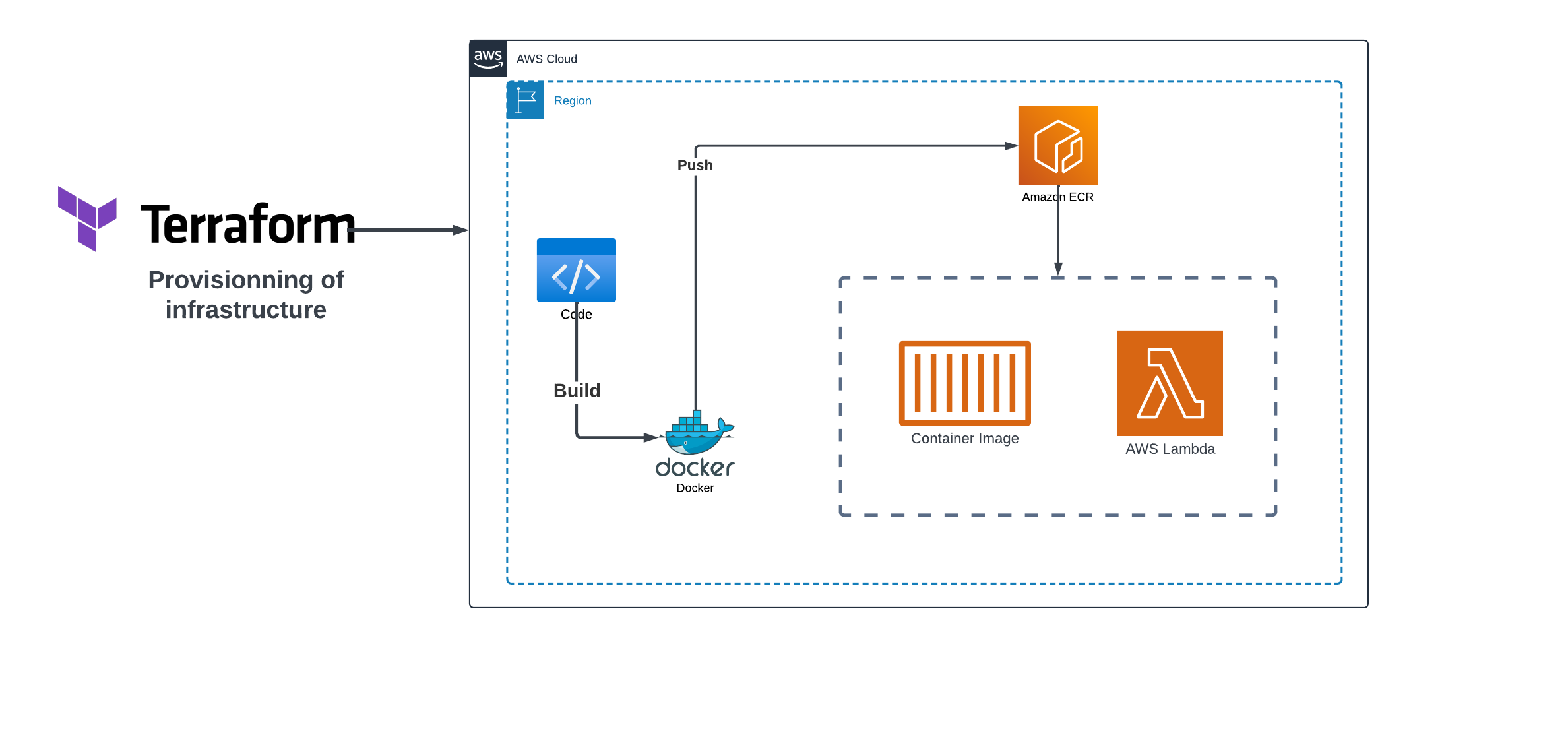
Creating a Containerized Lambda Function with Terraform
In the dynamic landscape of cloud computing, the fusion of containerization and serverless architecture is redefining how applications are developed and deployed. This article takes you on a concise yet comprehensive journey, demonstrating how to containerize a Python application, store it in Amazon ECR, and seamlessly deploy it as an AWS Lambda function using Terraform. Join us as we unravel the simplicity and power of this integration, offering a streamlined approach to building scalable and efficient cloud solutions.
Prerequisites
Before we begin, you should have the following prerequisites in place:
- An AWS account with appropriate permissions.
- Terraform and Docker installed on your local machine.
- AWS CLI installed and configured with your AWS credentials.
Containerizing and Pushing to Amazon ECR
In this section, we will walk through the process of containerizing a Python application using Docker, setting up an Amazon Elastic Container Registry (ECR) on AWS, and pushing the container image to ECR.
Containerizing a Python Application with Docker
To containerize a Python application, we’ll leverage Docker and create a Dockerfile. Here’s the source code of our Python application:
app.py
import json
def lambda_handler(event, context):
print(event)
print(context)
return {
'statusCode': 200,
'body': json.dumps('Hello World!!')
}
Dockerfile
FROM public.ecr.aws/lambda/python:3.8
COPY requirements.txt .
RUN pip3 install -r requirements.txt --target "${LAMBDA_TASK_ROOT}"
COPY . ${LAMBDA_TASK_ROOT}
CMD ["app.lambda_handler"]
requirements.txt
requests
This Dockerfile is tailored for AWS Lambda. It uses the official Lambda base image for Python, installs the required Python packages specified in requirements.txt
, and sets the entry point to our Python application.
Setting up Amazon Elastic Container Registry (ECR) on AWS
Before pushing the container image, let’s set up an Amazon ECR repository:
-
Open the AWS Management Console: Navigate to the AWS Management Console.
-
Go to Amazon ECR: In the services, find and click on Repositories under Private registry.
- Create a Repository:
- Click on Create repository.
- Provide a name for your repository (e.g.,
python-lambda-app
). - Optionally, add a tag to your repository.
- Click
Create repository
.
Pushing the Container Image to Amazon ECR
Now that we have our Dockerized Python application and an ECR repository set up, let’s push the container image to ECR:
-
Authenticate Docker to the ECR registry: Open your terminal and run the following command to authenticate Docker to your ECR registry.
aws ecr get-login-password --region <your-region> | docker login --username AWS --password-stdin <your-account-id>.dkr.ecr.<your-region>.amazonaws.com
-
Build and tag the Docker image: Navigate to the directory containing your Dockerfile and run the following commands:
docker build -t python-lambda-app . docker tag python-lambda-app:latest <your-account-id>.dkr.ecr.<your-region>.amazonaws.com/python-lambda-app:latest
Ensure to replace
<your-account-id>
and<your-region>
with your AWS account ID and the AWS region, respectively. -
Push the Docker image to ECR:
docker push <your-account-id>.dkr.ecr.<your-region>.amazonaws.com/python-lambda-app:latest
With these steps completed, your Python application is now containerized, and the container image is stored in your Amazon ECR repository. This sets the stage for the subsequent creation of an AWS Lambda function using Terraform.
Creating an AWS Lambda Function with Terraform
In this section, we will delve into the process of creating an AWS Lambda function using Terraform. This involves introducing Terraform and its role in Infrastructure as Code (IaC), crafting Terraform code to define the Lambda function, and configuring the function to utilize the container image stored in Amazon Elastic Container Registry (ECR).
Writing Terraform Code for Lambda Function
Let’s explore the Terraform code responsible for defining the AWS Lambda function:
main.tf
resource "aws_iam_role" "lambda_role" {
name = "iam_for_lambda"
assume_role_policy = jsonencode({
"Version" : "2012-10-17",
"Statement" : [{
"Action" : "sts:AssumeRole",
"Principal" : {
"Service" : "lambda.amazonaws.com"
},
"Effect" : "Allow"
}]
})
}
resource "aws_iam_policy" "iam_policy_for_lambda" {
name = "aws_iam_policy_lambda_role"
policy = jsonencode({
Version = "2012-10-17"
Statement = [
{
Effect = "Allow",
Action = [
"lambda:InvokeFunction",
"lambda:GetFunctionConfiguration",
],
Resource = "*"
}
]
})
}
resource "aws_iam_role_policy_attachment" "attach_iam_policy_to_iam_role" {
role = aws_iam_role.lambda_role.name
policy_arn = aws_iam_policy.iam_policy_for_lambda.arn
}
resource "aws_lambda_function" "python-lambda-function" {
function_name = "python-lambda-function"
description = "lambda function from terraform"
image_uri = var.image_uri
package_type = "Image"
architectures = ["x86_64"]
role = aws_iam_role.lambda_role.arn
}
provider.tf
provider "aws" {
region = "eu-north-1"
}
terraform {
required_providers {
aws = {
source = "hashicorp/aws"
version = "4.65.0"
}
}
}
variable.tf
variable "image_uri" {
default = "<your-account-id>.dkr.ecr.<your-region>.amazonaws.com/python-lambda-app:latest"
}
Configuring Lambda Function to Use ECR Container Image
Here’s a breakdown of the key components in the Terraform code:
-
IAM Role and Policy: Defines an IAM role for the Lambda function with a policy allowing essential Lambda actions.
-
Lambda Function Resource: Specifies the Lambda function’s details, including the name, description, container image URI, and IAM role.
-
Provider Configuration: Sets up the AWS provider with the desired region.
- Variables: Introduces a variable for the container image URI, providing flexibility in specifying the ECR image.
Deploying the Lambda Function
To deploy the Lambda function, execute the following Terraform commands in your terminal:
terraform init
terraform apply -auto-approve
This will initialize Terraform and apply the defined configuration, resulting in the creation of the Lambda function and associated IAM roles.
Testing
Now that we have created our Lambda function using Terraform, let’s conduct testing to ensure its successful integration. Follow these steps to execute Terraform commands, verify deployment, and perform testing:
Testing the Integrated Solution
-
Access AWS Management Console: Open the AWS Management Console.
-
Navigate to Lambda Service: In the console, navigate to the Lambda service.
- Select Your Lambda Function: Find and select the Lambda function named
python-lambda-function
or the name you specified.
-
Invoke the Lambda Function: Within the Lambda function details page, locate the "Test" button. Click on it to manually invoke the Lambda function.
- Review Execution Results: After invocation, review the execution results, including any logs or outputs generated by the Lambda function.
Conclusion
This tutorial showcased the streamlined process of creating a containerized AWS Lambda function using Terraform and Amazon ECR. From Dockerizing a Python application to defining infrastructure as code (IaC) with Terraform, we demonstrated a cohesive workflow for efficient deployment. The combination of containerization, Terraform, and AWS services offers flexibility and scalability, laying the groundwork for diverse serverless applications. As you delve into this integration, explore customization possibilities and leverage the power of orchestrated serverless solutions for your specific needs. Happy coding!
Leave a Reply